Understanding Controller Properties
Display panels come in all types, shapes, and sizes. Tibbo supports a limited but growing number of controllers/panels. Each supported controller requires its own "mini-driver." Your application cannot specify the desired controller. Instead, the controller type is selected through the Customize Platform dialog, accessible through the Project Settings dialog. Once this is done, a proper "mini-driver" is added to the executable binary during compilation.
The lcd. object is ready to work with a wide range of displays, some of which are not actually of the LCD type. So, the proper name for this object should be "display object." Nevertheless, we have decided to keep this name — typing "lcd" is faster and the name has been in use for a while.
A number of read-only properties inform your application about the type and other vital parameters of the currently selected display:
- lcd.paneltype indicates whether the panel is of monochrome/grayscale or color type.
- lcd.bitsperpixel tells you how many bits in the display controller's memory are allocated for each display pixel. Of course, the more bits/pixel you get, the higher the display quality.
- For displays with 1, 2, or 4 bits/pixel, a single byte of memory packs the data for 8, 4, or 2 pixels. The lcd.pixelpacking property will inform you how pixels are packed into memory bytes: vertically, or horizontally (see the drawing below). The reason you may want to know this is to achieve faster text output.
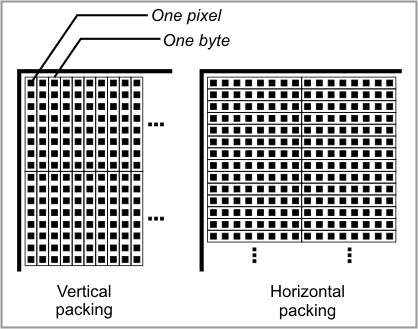
Color Displays
For color displays (lcd.paneltype = 1 — PL_LCD_PANELTYPE_COLOR), three additional read-only properties — lcd.redbits, lcd.greenbits, and lcd.bluebits — will indicate how many bits are available in each color channel, and also how three color fields are combined into a word describing the overall color of the pixel. You need to know this for setting pixels, as well as defining the foreground/background color used in drawing lines and rectangles, filling areas, and printing text (see Working With Pixels and Colors).
These three properties are of word type. Each 16-bit value packs two 8-bit parameters: number of bits per pixel for this color channel (high byte) and the bit position of the field in the color word (low byte). For example, if lcd.redbits = &h0500, lcd.bluebits = &h0605, and lcd.greenbits = &h050B, you reconstruct the composition of the red, green, and blue bits in a word:

In this example, the red field is the first one on the right, followed by the blue field (this field starts from bit 5) and the green field (starts from bit 11 or 7hB). You now also know that there are 52 = 32 brightness levels for red and green, and 64 brightness levels for blue.
You can use this detailed information to select color values that will work correctly on all color displays, even those you haven't tested yet. Here is a useful example where you work out three constants — color_red, color_green, and color_blue — that will universally work for any color display.
Dim color_red, color_green, color_blue As word
...
lcd_red=val("&b"+strgen(lcd.redbits/&hFF,"1")+strgen(lcd.redbits And &hFF,"0"))
lcd_green=val("&b"+strgen(lcd.greenbits/&hFF,"1")+strgen(lcd.greenbits And &hFF,"0"))
lcd_blue=val("&b"+strgen(lcd.bluebits/&hFF,"1")+strgen(lcd.bluebits And &hFF,"0"))
Now you can scientifically work out the constant for the white color:
...
lcd_white=lcd_red+lcd_green+lcd_blue
In reality, you don't have to bother calculating color_white like this. Just select the highest possible value (&hFFFF) and this will be your white.