Working With Text
The lcd.print and lcd.printaligned methods display text. The text is printed using the selected font. This means you need to have at least one font file in your project (see how to add a file), and have this font selected before you can print anything.
The lcd.setfont method is used to select the font:
romfile.open("Tibbo-5x7(VP).bin")
lcd.setfont(romfile.offset)
Note that lcd.setfont will return 1 — NG if you try to feed it a wrong file!
Once the font has been successfully selected, the lcd.fontheight and lcd.fontpixelpacking read-only properties will tell you the maximum height of characters in this font and how pixel data is packed within the font file. The meaning of the first one is obvious. The meaning of the second one will become apparent after (and if) you read Raster Font File Format. If you don't want to read this, it's OK — we can just go straight to the summary:
Fonts can be encoded horizontally or vertically, and lcd.fontpixelpacking will tell you what type of font you have selected. You should only care about this for displays with lcd.bitsperpixel < 8. if your display has lcd.bitsperpixel > 8, then it doesn't matter what kind of font you use. If it is < 8, then you are better off selecting a font for which lcd.fontpixelpacking is equal to lcd.pixelpacking (depends on the selected controller/panel). You achieve better performance when these two properties are "aligned." It will also work if you select a font which is "perpendicular" to your display, but text printing might slow down considerably.
We typically offer two versions for each font; for example, "Tibbo-5x7(V).bin" and "Tibbo-5x7(H)" — V stands for "vertical" and "H" stands "horizontal."
Non-Aligned Text
Once the font has been selected, you can start printing. You do this with the lcd.print method. This method always produces single-line output. Two properties — lcd.textorientation and lcd.texthorizontalspacing — affect how your text is printed. The reference point of your text is at the top-left pixel of the output. The x and y arguments of lcd.print specify this corner, and the text is rotated "around" this pixel as well. For example:
lcd.print("Text",30,10)
lcd.textorientation=PL_LCD_TEXT_ORIENTATION_180
lcd.texthorizontalspacing=4
lcd.print("Text",30,10)
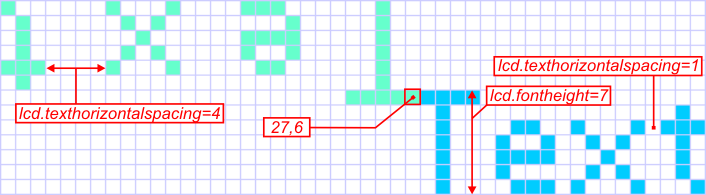
The lcd.print method returns the total width of produced textual output in pixels. This can be very useful. Here is an example where you draw a frame around the text, and you want the frame size to be "just right":
x=lcd.print("Text",30,10)
lcd.rectangle(28,8,30+x+1,10+lcd.fontheight+1)
Sometimes, it is desirable to know the width of the text output before actual printing. The lcd.getprintwidth method will tell you how many pixels will be taken by your text horizontally (remember, text height is equal to lcd.fontheight). In the following example, we align the text output along the right side of the screen. This requires us to know how wide this output will be:
x=lcd.getprintwidth("Text")
x=lcd.print("Text",lcd.width-x,10)
Aligned Text
The lcd.printaligned method outputs your text within a specified rectangular area. Four properties — lcd.textorientation, lcd.textalignment, lcd.texthorizontalspacing, and lcd.textverticalspacing — define how your text will be printed. To fit within the target area, the lcd. object will split the text into several lines as necessary. Only the text that can fit within the area will be displayed. You can add your own line breaks by using the ` character (ASCII code 96). For example:
lcd.textverticalspacing=2
lcd.textalignment=PL_LCD_TEXT_ALIGNMENT_BOTTOM_RIGHT
lcd.printaligned("Multi-line text",0,0,35,33)
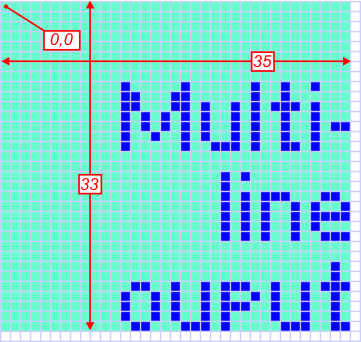
Note that lcd.printaligned returns the number of text lines that were produced.