Direct Sector Access
Discussed in this section: fd.buffernum, fd.getbuffer, fd.setbuffer, fd.getsector, fd.setsector, fd.checksum, fd.copyfirmware. fd.copyfirmwarelzo.
Direct sector access allows you to work with sectors in the data area of your device's flash memory directly, without the need to create and manage any files. You work with sectors through two identical 264-byte (platform-specific) RAM buffers numbered #0 and #1. The fd.buffernum property selects one of the buffers as the source/destination for the data (see the drawing below).
All file-based operations of the flash disk also rely on the RAM buffers and the selected buffer number may change as a result of their execution. When using direct sector access and file-based access concurrently, switch to RAM buffer #0 each time before performing direct sector access — this will guarantee that you won't corrupt the files and/or the file system and cause disk dismounting (fd.ready becoming 0 — NO). There is more on this in the File-Based and Direct Sector Coexistence topic.
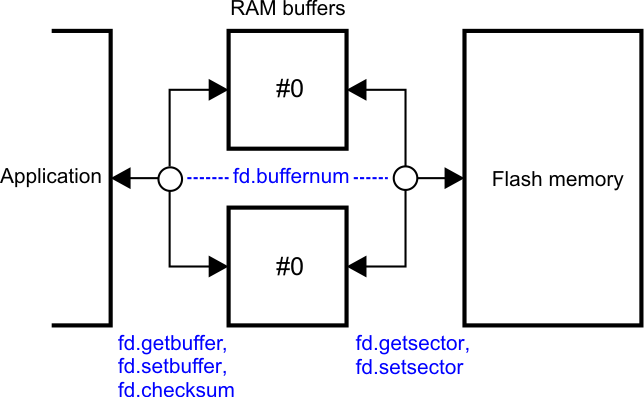
Reading data from a sector is a two-step process. First, you use fd.getsector to load all 264 bytes from the desired sector into the currently selected RAM buffer. Next, you use fd.getbuffer to read the data from the selected buffer. This method allows you to read up to 255 bytes beginning from any offset in the buffer (offsets are counted from 0):
Dim s As String
...
'we want bytes 20-29 from sector #3
fd.buffernum=0 'select RAM buffer #0
If fd.getsector(3)<>PL_FD_STATUS_OK Then
'flash failure
End If
s=fd.getbuffer(20,10) 'now s contains the desired data
Since the sector and (RAM buffer size) exceeds 255 bytes (maximum length of string variables), you can't actually read the whole sector contents in one portion. At least two fd.getbuffer reads are necessary for that.
To modify the data in the selected RAM buffer, use the fd.setbuffer method, which allows you to write new data at any offset of the selected RAM buffer. To store the contents of the RAM buffer back to the flash memory, use fd.setsector:
Dim s As String
...
'modify first 3 bytes of sector #5
If fd.getsector(3)<>PL_FD_STATUS_OK Then
'flash failure
End If
fd.setbuffer("ABC",0) 'write "ABC" to the buffer at offset 0
If fd.setsector(3)<>PL_FD_STATUS_OK Then
'flash failure
End If
Since there are two identical RAM buffers, you can load the contents of two different sectors and work with the data concurrently by switching between the buffers.
As covered under Sharing Flash Between Your Application and Data, logical sector numbers for fd.getsector and fd.setsector are not actual physical sector numbers of the flash IC. Logical sector #0 corresponds to the topmost physical sector of the flash IC, so the logical numbering is "in reverse."
You can only write to sectors that reside within the data area of your flash chip (that is, logical sector numbers from 0 to fd.availableflashspace - 1). For devices with shared flash memory, this prevents your application from inadvertently damaging its own code or firmware. Trespassing the data area boundary will result in the 4 — PL_FD_STATUS_INV_PARAM status code. If you want to alter the data in the firmware/application area, see Self-Upgrading the Firmware/Application.
If you are using direct sector access and file-based access at the same time, be sure to read about ensuring their proper coexistence.
Note also that fd.getsector and fd.setsector always access the actual specified target sector and not its cached copy, even if a disk transaction is in progress (fd.transactionstarted = 1 — YES) and the target sector has been cached already.